Scene Resources¶
Components required to construct a 3D scene with views of Element
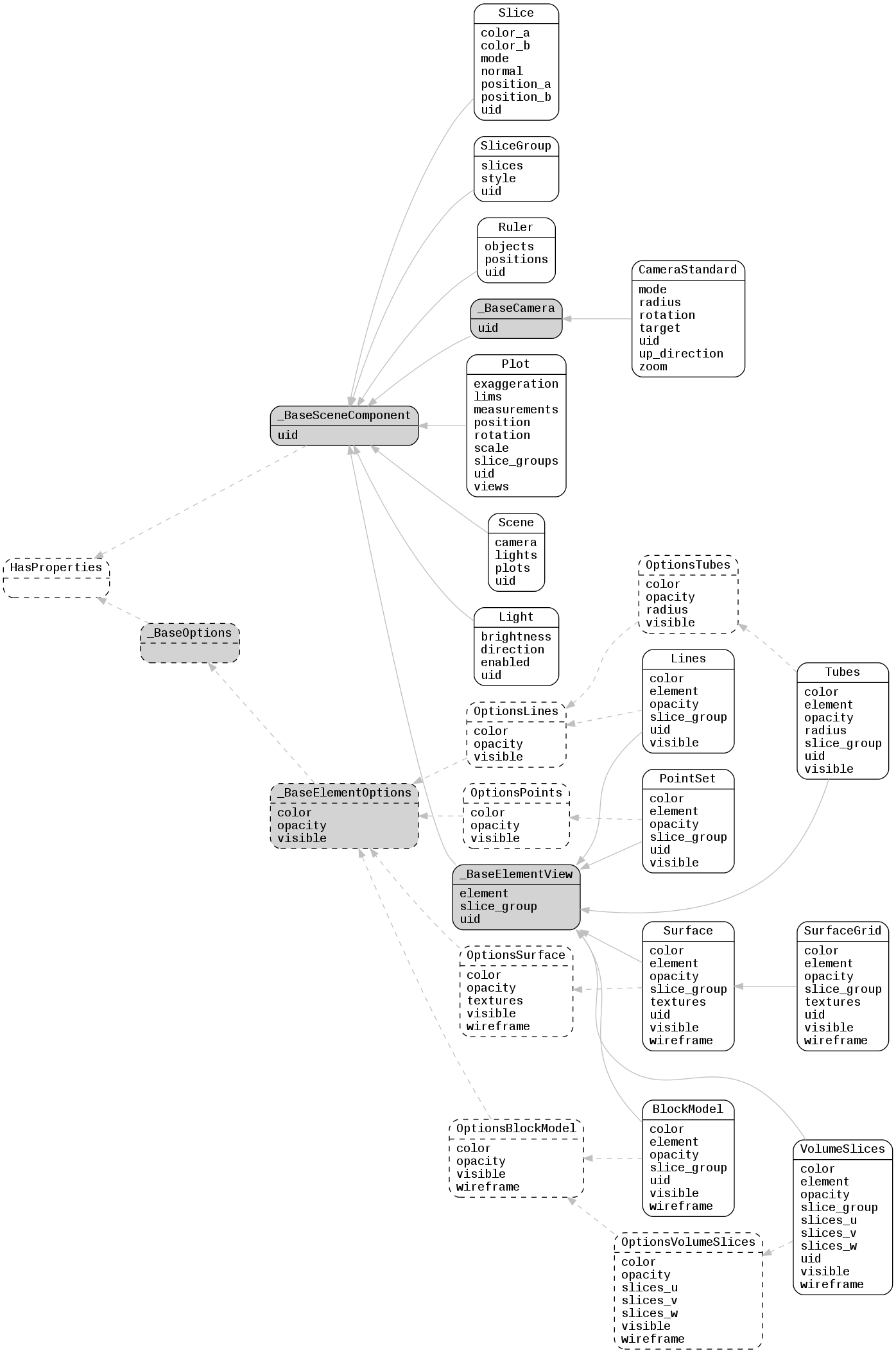
Doc links:
_BaseCamera
_BaseElementOptions
_BaseElementView
_BaseOptions
_BaseSceneComponent
BlockModel
CameraStandard
HasProperties
Light
Lines
OptionsBlockModel
OptionsLines
OptionsPoints
OptionsSurface
OptionsTubes
OptionsVolumeSlices
Plot
PointSet
Ruler
Scene
Slice
SliceGroup
Surface
SurfaceGrid
Tubes
VolumeSlices
-
class
lfview.resources.scene.scene.
_BaseSceneComponent
(**kwargs)¶ Base class for the Scene and all objects within a scene
Optional Properties:
- uid (
String
): Locally unique ID from client, a unicode string
- uid (
-
class
lfview.resources.scene.scene.
Light
(**kwargs)¶ Light source for a scene
Required Properties:
- brightness (
Float
): Intensity of light source, a float - direction (
Vector3
): Vector pointing from plot center to light, a 3D Vector of <class ‘float’> with shape (3) - enabled (
Boolean
): Whether light is on or off, a boolean
Optional Properties:
- uid (
String
): Locally unique ID from client, a unicode string
- brightness (
-
class
lfview.resources.scene.scene.
Slice
(**kwargs)¶ Position and color information for a slice in the Scene
Required Properties:
- color_a (
Color
): Color of first slice, a color, Default: [0, 120, 255] - mode (
StringChoice
): Slice mode, any of “inactive” (Slice is currently disabled), “normal” (Standard single slice slicing mode), “reversed” (Single slice with swapped normal), “section” (Mode with two parallel sliceplanes), Default: inactive - normal (
Vector3
): Normal vector to the slice plane, a 3D Vector of <class ‘float’> with shape (3), Default: new instance of list - position_a (
Vector3
): Position of first plane, a 3D Vector of <class ‘float’> with shape (3), Default: new instance of list
Optional Properties:
- color_b (
Color
): Color of second slice; only required in section mode, a color, Default: [253, 102, 0] - position_b (
Vector3
): Position of second plane; only required in section mode, a 3D Vector of <class ‘float’> with shape (3), Default: [0, 0, 0] - uid (
String
): Locally unique ID from client, a unicode string
- color_a (
-
class
lfview.resources.scene.scene.
SliceGroup
(**kwargs)¶ Class describing a group of slices
These slice the same views in the same style (union vs intersection)Required Properties:
- slices (a list of
Slice
): Slices in this group; only one is currently supported, a list (each item is an instance of Slice) with length of 1, Default: new instance of list - style (
StringChoice
): How slices in this group are combined, either “union” or “intersection”, Default: intersection
Optional Properties:
- uid (
String
): Locally unique ID from client, a unicode string
- slices (a list of
-
class
lfview.resources.scene.scene.
Ruler
(**kwargs)¶ Ruler class
Contains the path of the ruler as well as info about the objects it is measuring to and fromRequired Properties:
- objects (a list of
_BaseElement
instance or UID): URL pointers of objects we are measuring from/to, a list (each item is an instance of _BaseElement or a valid uid property of that class) with length of 2 - positions (a list of
Vector3
): Endpoints of the ruler, a list (each item is a 3D Vector of <class ‘float’> with shape (3)) with length of 2
Optional Properties:
- uid (
String
): Locally unique ID from client, a unicode string
- objects (a list of
-
class
lfview.resources.scene.scene.
_BaseCamera
(**kwargs)¶ Base Camera class from which all cameras must inherit
Optional Properties:
- uid (
String
): Locally unique ID from client, a unicode string
- uid (
-
class
lfview.resources.scene.scene.
CameraStandard
(**kwargs)¶ Base class for Standard Cameras
Both orthographic and perspective cameras are built on thisRequired Properties:
- mode (
StringChoice
): View mode of camera, either “perspective” or “orthographic”, Default: orthographic - radius (
Float
): Distance of camera to target, a float, Default: 5.0 - rotation (a list of
Float
): Quaternion rotation of the camera relative to the scene, a list (each item is a float) with length of 4, Default: new instance of list - target (
Vector3
): Center of rotation of camera relative to the scene origin, a 3D Vector of <class ‘float’> with shape (3), Default: new instance of list - up_direction (
Vector3
): Up direction of camera, a 3D Vector of <class ‘float’> with shape (3), Default: up - zoom (
Float
): Zoom level of camera, a float, Default: 1.0
Optional Properties:
- uid (
String
): Locally unique ID from client, a unicode string
- mode (
-
class
lfview.resources.scene.scene.
_BaseElementView
(**kwargs)¶ Base View class for all views to inherit from
Contains basic meta properties (color, opacity, visible) and supports data mapping to the opacity and color attributesRequired Properties:
- element (
_BaseElement
instance or UID): URL pointer of element associated with this view, an instance of _BaseElement or a valid uid property of that class
Optional Properties:
- element (
-
class
lfview.resources.scene.scene.
PointSet
(**kwargs)¶ PointSetView class, used by PointSet Element
Supports data mapping to the point size attributeRequired Properties:
- color (
OptionsColor
): Default color options on the element, an instance of OptionsColor, Default: new instance of OptionsColor - element (
ElementPointSet
instance or UID): URL pointer of element associated with this view, an instance of ElementPointSet or a valid uid property of that class - opacity (
OptionsOpacity
): Default opacity options on the element, an instance of OptionsOpacity, Default: new instance of OptionsOpacity - visible (
Boolean
): Visibility of resource on/off, a boolean, Default: True
Optional Properties:
- color (
-
class
lfview.resources.scene.scene.
Lines
(**kwargs)¶ LineSetView class, used by LineSet Element
Required Properties:
- color (
OptionsColor
): Default color options on the element, an instance of OptionsColor, Default: new instance of OptionsColor - element (
ElementLineSet
instance or UID): URL pointer of element associated with this view, an instance of ElementLineSet or a valid uid property of that class - opacity (
OptionsOpacity
): Default opacity options on the element, an instance of OptionsOpacity, Default: new instance of OptionsOpacity - visible (
Boolean
): Visibility of resource on/off, a boolean, Default: True
Optional Properties:
- color (
-
class
lfview.resources.scene.scene.
Tubes
(**kwargs)¶ TubesView class, used by LineSet Element
Supports data mapping to the radius attributeRequired Properties:
- color (
OptionsColor
): Default color options on the element, an instance of OptionsColor, Default: new instance of OptionsColor - element (
ElementLineSet
instance or UID): URL pointer of element associated with this view, an instance of ElementLineSet or a valid uid property of that class - opacity (
OptionsOpacity
): Default opacity options on the element, an instance of OptionsOpacity, Default: new instance of OptionsOpacity - radius (
OptionsSize
): Default opacity options on the element, an instance of OptionsSize, Default: new instance of OptionsSize - visible (
Boolean
): Visibility of resource on/off, a boolean, Default: True
Optional Properties:
- color (
-
class
lfview.resources.scene.scene.
Surface
(**kwargs)¶ SurfaceView class, used by SurfaceElement
Required Properties:
- color (
OptionsSurfaceColor
): Default color options on the element, an instance of OptionsSurfaceColor, Default: new instance of OptionsSurfaceColor - element (
ElementSurface
instance or UID): URL pointer of element associated with this view, an instance of ElementSurface or a valid uid property of that class - opacity (
OptionsOpacity
): Default opacity options on the element, an instance of OptionsOpacity, Default: new instance of OptionsOpacity - textures (a list of
OptionsTexture
): Default textures on the element, a list (each item is an instance of OptionsTexture) - visible (
Boolean
): Visibility of resource on/off, a boolean, Default: True - wireframe (
OptionsWireframe
): Default wireframe options on the element, an instance of OptionsWireframe, Default: new instance of OptionsWireframe
Optional Properties:
- color (
-
class
lfview.resources.scene.scene.
SurfaceGrid
(**kwargs)¶ SurfaceGridView class, used by element with SurfaceGrid geometry
Required Properties:
- color (
OptionsSurfaceColor
): Default color options on the element, an instance of OptionsSurfaceColor, Default: new instance of OptionsSurfaceColor - element (
ElementSurfaceGrid
instance or UID): URL pointer of element associated with this view, an instance of ElementSurfaceGrid or a valid uid property of that class - opacity (
OptionsOpacity
): Default opacity options on the element, an instance of OptionsOpacity, Default: new instance of OptionsOpacity - textures (a list of
OptionsTexture
): Default textures on the element, a list (each item is an instance of OptionsTexture) - visible (
Boolean
): Visibility of resource on/off, a boolean, Default: True - wireframe (
OptionsWireframe
): Default wireframe options on the element, an instance of OptionsWireframe, Default: new instance of OptionsWireframe
Optional Properties:
- color (
-
class
lfview.resources.scene.scene.
BlockModel
(**kwargs)¶ BlockModelView class, used by Volume Element
Required Properties:
- color (
OptionsColor
): Default color options on the element, an instance of OptionsColor, Default: new instance of OptionsColor - element (
ElementVolumeGrid
instance or UID): URL pointer of element associated with this view, an instance of ElementVolumeGrid or a valid uid property of that class - opacity (
OptionsOpacity
): Default opacity options on the element, an instance of OptionsOpacity, Default: new instance of OptionsOpacity - visible (
Boolean
): Visibility of resource on/off, a boolean, Default: True - wireframe (
OptionsWireframe
): Default wireframe options on the element, an instance of OptionsWireframe, Default: new instance of OptionsWireframe
Optional Properties:
- color (
-
class
lfview.resources.scene.scene.
VolumeSlices
(**kwargs)¶ VolumeSlicesView class, used by Volume Element
Required Properties:
- color (
OptionsColor
): Default color options on the element, an instance of OptionsColor, Default: new instance of OptionsColor - element (
ElementVolumeGrid
instance or UID): URL pointer of element associated with this view, an instance of ElementVolumeGrid or a valid uid property of that class - opacity (
OptionsOpacity
): Default opacity options on the element, an instance of OptionsOpacity, Default: new instance of OptionsOpacity - slices_u (a list of
Float
): List of active slice locations along u axis, a list (each item is a float in range [0.0, 1.0]) with length between 0 and 256, Default: new instance of list - slices_v (a list of
Float
): List of active slice locations along v axis, a list (each item is a float in range [0.0, 1.0]) with length between 0 and 256, Default: new instance of list - slices_w (a list of
Float
): List of active slice locations along w axis, a list (each item is a float in range [0.0, 1.0]) with length between 0 and 256, Default: new instance of list - visible (
Boolean
): Visibility of resource on/off, a boolean, Default: True - wireframe (
OptionsWireframe
): Default wireframe options on the element, an instance of OptionsWireframe, Default: new instance of OptionsWireframe
Optional Properties:
- color (
-
class
lfview.resources.scene.scene.
Plot
(**kwargs)¶ Object describing the contents of the 3D scene
Contains info about how a plot is positioned in the scene, as well as the plot limits. Also contains active elements, slices, and measurements in the plot.Required Properties:
- exaggeration (a list of
Integer
): x,y,z exaggeration of the plot coordinates, a list (each item is an integer in range [1, inf]), Default: new instance of list - lims (a list of
Vector2
): x,y,z limits, defined in plot coordinates, a list (each item is a 2D Vector of <class ‘float’> with shape (2)) with length of 3, Default: new instance of list - measurements (a list of
Ruler
): List of active ruler instances; currently only one is supported, a list (each item is an instance of Ruler) with length between 0 and 1 - position (
Vector3
): x,y,z position of the plot center relative to scene origin, a 3D Vector of <class ‘float’> with shape (3), Default: new instance of list - rotation (a list of
Float
): Quaternion rotation of plot axes relative to scene axes, a list (each item is a float) with length of 4, Default: new instance of list - scale (
Vector3
): x,y,z scale of the plot coordinate system relative to scene coordinate system, a 3D Vector of <class ‘float’> with shape (3), Default: new instance of list - slice_groups (a list of
SliceGroup
): Active slice groups; currently only one is supported, a list (each item is an instance of SliceGroup) with length between 0 and 1, Default: new instance of list - views (a list of
PointSet
, a list ofLines
, a list ofTubes
, a list ofSurface
, a list ofSurfaceGrid
, a list ofBlockModel
, a list ofVolumeSlices
): List of element views in the plot, a list (each item is an instance of PointSet or an instance of Lines or an instance of Tubes or an instance of Surface or an instance of SurfaceGrid or an instance of BlockModel or an instance of VolumeSlices) with length between 0 and 100
Optional Properties:
- uid (
String
): Locally unique ID from client, a unicode string
- exaggeration (a list of
-
class
lfview.resources.scene.scene.
Scene
(**kwargs)¶ State of the 3D scene
Includes camera, lighting, and plot infoRequired Properties:
- camera (
CameraStandard
): Scene camera, an instance of CameraStandard, Default: new instance of CameraStandard - lights (a list of
Light
): Active lights, a list (each item is an instance of Light) with length between 0 and 4 - plots (a list of
Plot
): Plots in the scene; currently only one is supported, a list (each item is an instance of Plot) with length between 0 and 1, Default: new instance of list
Optional Properties:
- uid (
String
): Locally unique ID from client, a unicode string
- camera (